需求说明:H5实现初始化通过高德地图进行定位城市,用户也可以自行点击选择其他城市
主要功能点:定位、城市选择器
实现效果
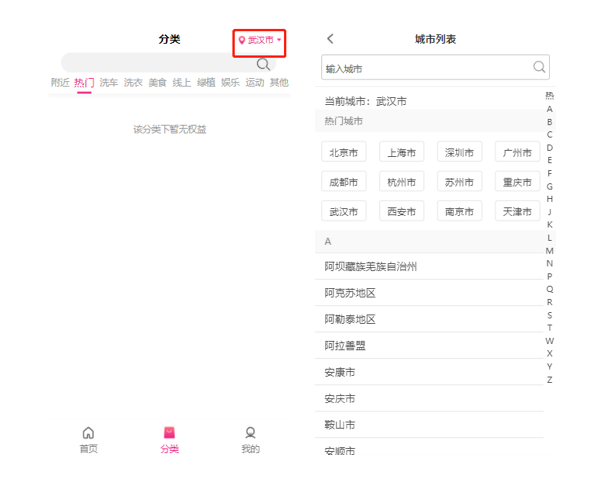
一、初始准备
这里采用 高德地图 JS API去实现上述两项功能,使用高德地图 JS API 开发地图应用需要先注册账号并申请Key,https://lbs.amap.com/api/javascript-api/guide/abc/prepare
首先,注册开发者账号,成为高德开放平台开发者,地址 https://console.amap.com/dev/id/choose
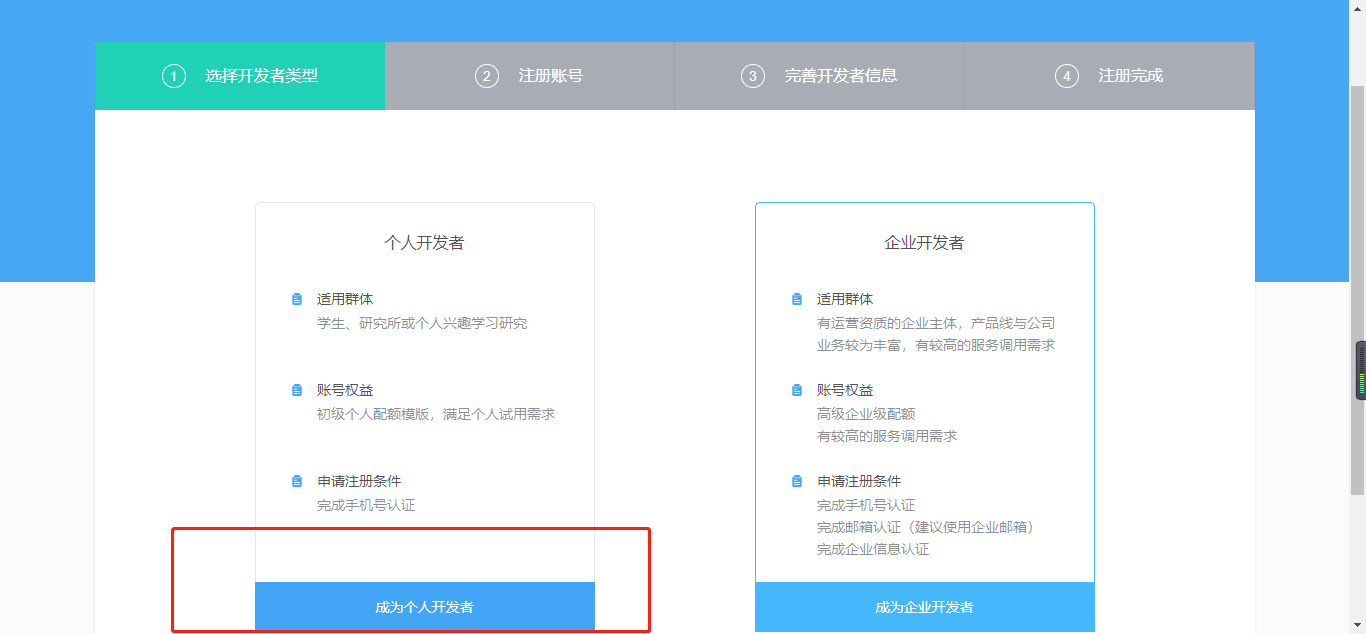
这里我选择的个人开发者,只需要手机号认证即可创建,后续有需求可以选择企业开发者。
登陆之后,在进入「应用管理」 页面「创建新应用」
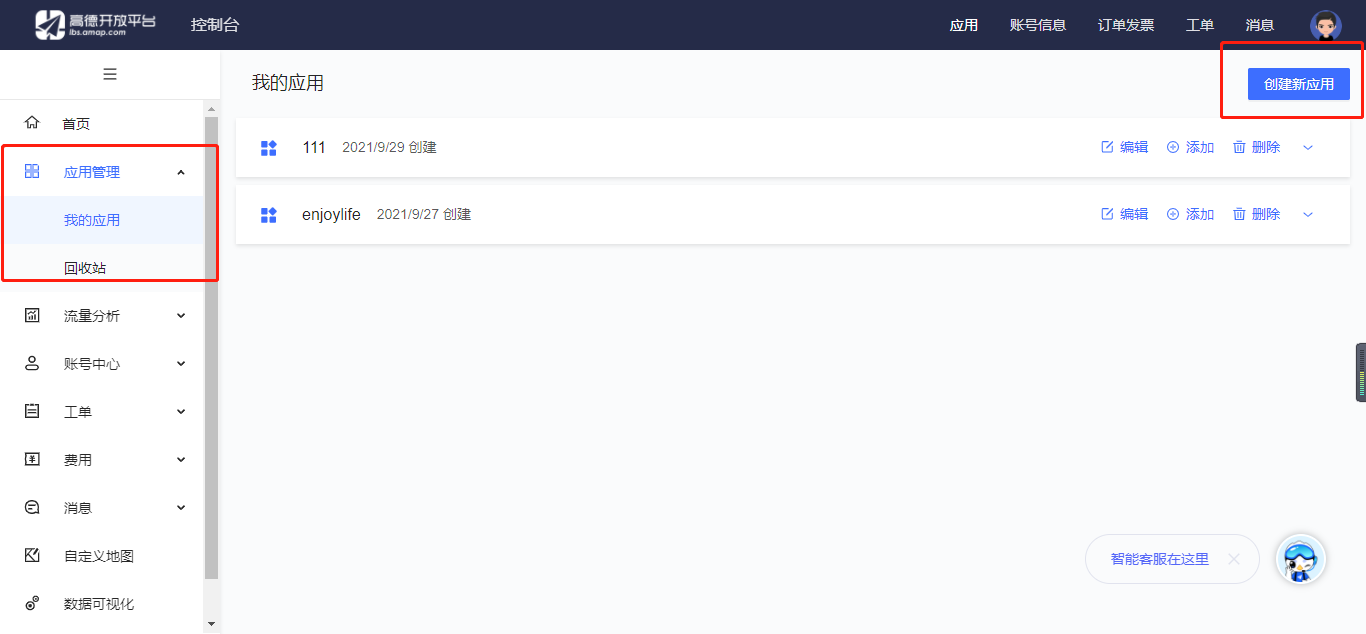
为应用添加 Key,「服务平台」一项选择「 Web 端 ( JSAPI ) 」
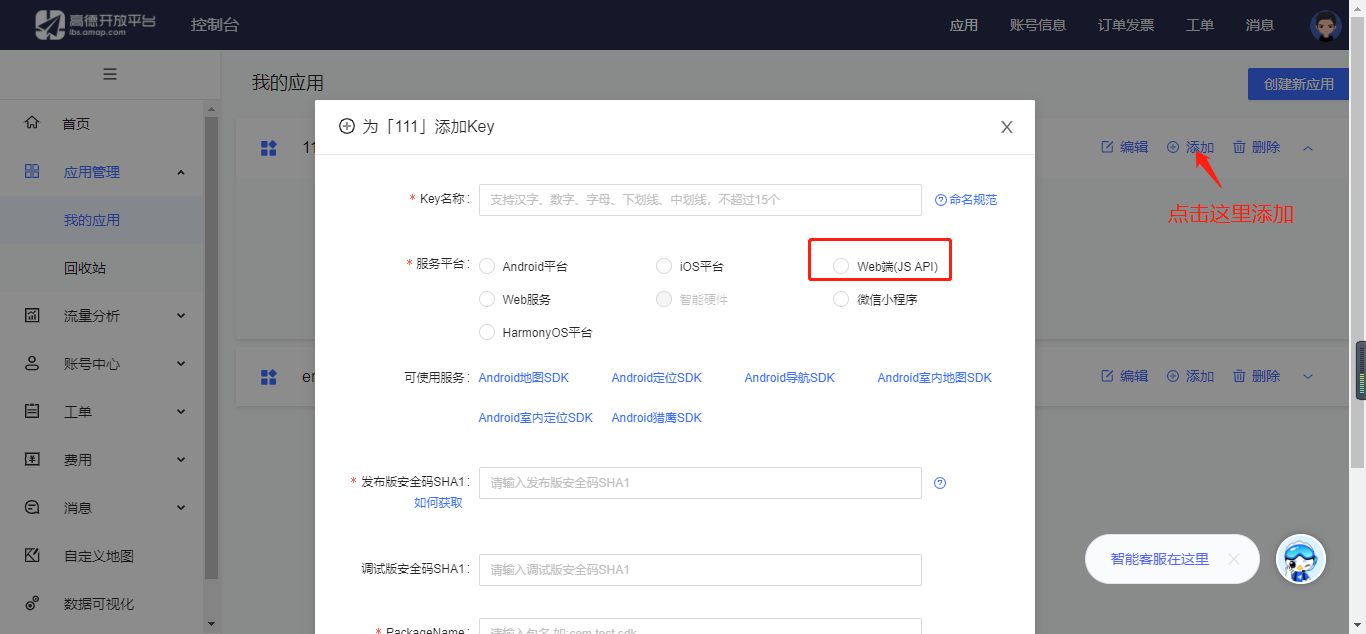
添加后点击可以查看新生成的key,将其复制添加到项目中
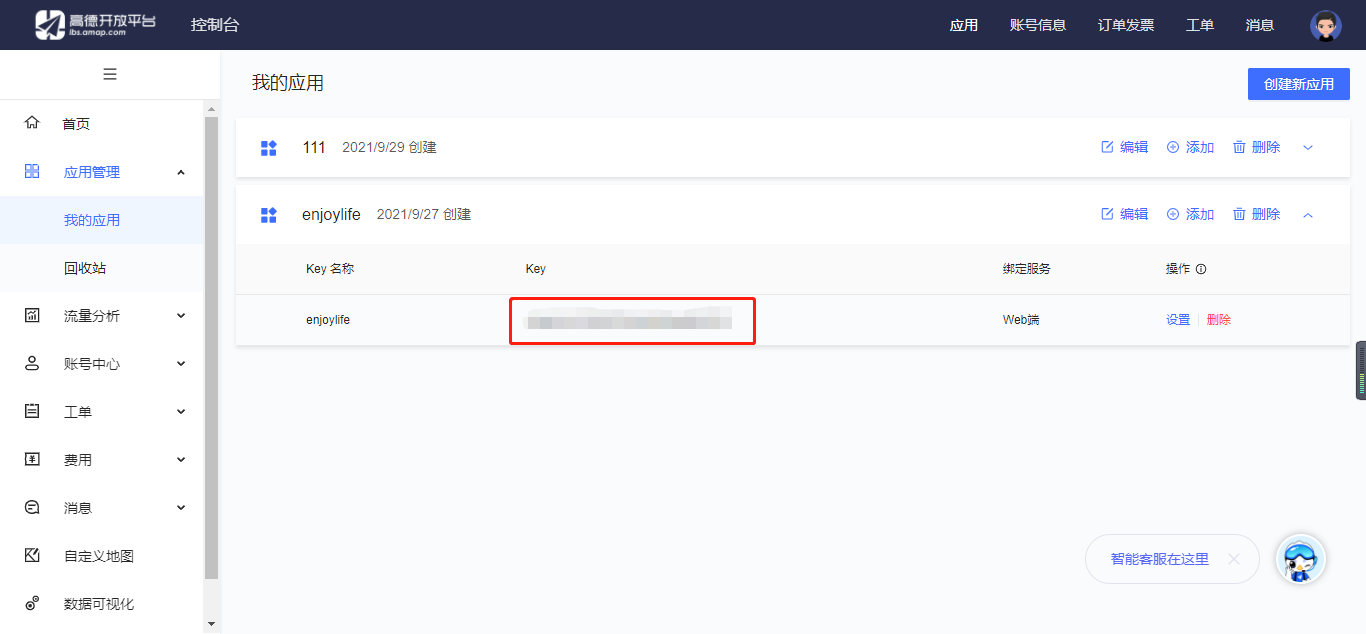
二、Vue项目初始配置
在main.html页面添加 JS API 的入口脚本标签,并将其中key值=刚刚申请的 key,由于还使用到高德的城市选择器组件,所以还需要引入ui组件库
1 2 3 4
| <!--引入高德地图JSAPI --> <script src="//webapi.amap.com/maps?v=2.0&key=申请的key"></script> <!--引入UI组件库(1.1版本) --> <script src="//webapi.amap.com/ui/1.1/main.js"></script>
|
在webpack.base.conf.js中加入
1 2 3 4 5 6
| module.exports = { externals: { 'AMap': 'AMap', 'AMapUI': 'AMapUI' }, }
|
三、定位功能实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77
| import AMap from 'AMap'; methods:{ getLocation() { const self = this AMap.plugin('AMap.Geolocation', function () { var geolocation = new AMap.Geolocation({ enableHighAccuracy: true, timeout: 10000, }) geolocation.getCityInfo(function(status,result){ if(status=='complete'){ self.cityName = result.city; self.cityCode = result.adcode; console.log(self.cityName,self.cityCode) }else{ onError(result) } }) geolocation.getCurrentPosition(function(status,result){ if(status=='complete'){ self.longitude = result.position.lng; self.latitude = result.position.lat; }else{ onError(result) } }); }); function onError(data) { self.getLngLatLocation(); } }, getLngLatLocation() { let self = this; AMap.plugin('AMap.CitySearch', function () { var citySearch = new AMap.CitySearch(); citySearch.getLocalCity(function (status, result) { if (status === 'complete' && result.info === 'OK') { console.log('通过ip获取当前城市:', result) self.cityName = result.city; self.cityCode = result.adcode; var lnglat = result.rectangle.split(';')[0].split(','); console.log(lnglat) self.longitude = lnglat[0]; self.latitude = lnglat[1]; AMap.plugin('AMap.Geocoder', function () { var geocoder = new AMap.Geocoder({ city: result.adcode }) var lnglat = result.rectangle.split(';')[0].split(','); geocoder.getAddress(lnglat, function (status, data) { if (status === 'complete' && data.info === 'OK') { console.log(data) } }) }) }else { } }) }) } }, mounted(){ this.getLocation(); }
|
四、城市选择器功能实现
官网 https://lbs.amap.com/api/amap-ui/reference-amap-ui/other/mobicitypicker
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| <template> <div id="container" class="mymap"></div> </template> <script>
import AMapUI from "AMapUI";
export default { data() { return { }; }, mounted() { var self = this; AMapUI.loadUI(["misc/MobiCityPicker"], function (MobiCityPicker) { var cityPicker = new MobiCityPicker({ theme: "red", }); cityPicker.on("citySelected", function (cityInfo) { cityPicker.hideImmediately(); console.log(cityInfo); }); cityPicker.show(); }); }, methods: {}, }; </script> <style scoped> .mymap { width: 100%; height: 100%; } </style>
|
五、城市选择器样式自定义
设置自定义主题
1 2 3 4
| var cityPicker = new MobiCityPicker({ theme: "red", });
|
在main.js中引入自定义css
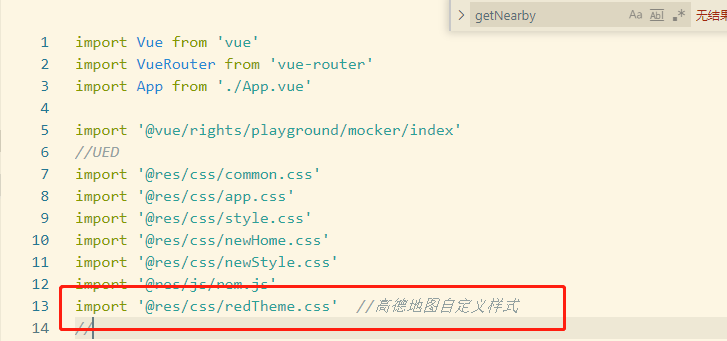
3.自定义样式css需要带上red
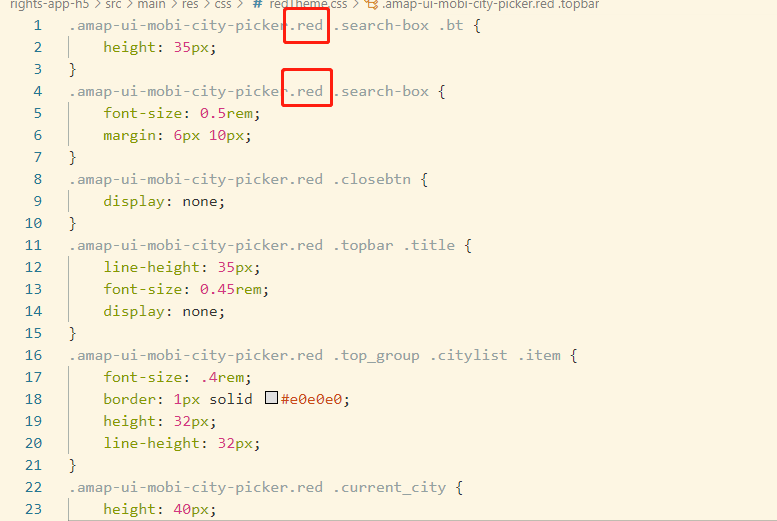